controllability and observability
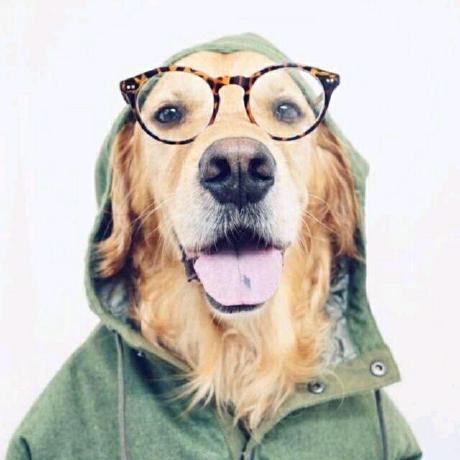
1 | from sympy import * |
Problem 4.1
Determine whether the following continuous-time linear time-invariant system is fully controllable
1 | A = Matrix([[0, 1, 0], |
This system is fully controllable
Problem 4.2
Determine the range of values a,b,c for the following continuous-time linear time-invariant systems to be fully controllable
1 | a, b= symbols("a b") |
1 | if Q.rank()==3: |
This system is not fully controllable
Problem 4.3
Determine whether the following continuous-time linear time-invariant system is fully observable
1 | A = Matrix([[0, 1, 0], |
This system is fully controllable
Problem 4.4
Determine the range of values a,b,c for the following continuous-time linear time-invariant system to be fully observable
Condition 1: a!=0 or b!=1
1 | # if a!=0 and b!=1: C.rank()=2, only when rank[C CA]'==3, the conditions are satified |
Condition 2: a==0 and b==1
1 | # only when rank[C1 C1A C1A^2]'==3, the conditions are satified |
rank(Q) = 3, thus in this condition, this system is not fully observable
Problem 4.5
Determine the range of values a,b,c for the following continuous-time linear time-invariant system to be fully controllable and observable
Problem 4.6
Calculate the controllability and observability index of the following continuous-time linear time-invariant system
- 标题: controllability and observability
- 作者: Oliver xu
- 创建于 : 2019-08-13 14:40:48
- 更新于 : 2025-07-18 21:08:09
- 链接: https://blog.oliverxu.cn/2019/08/13/controllability-and-observability/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。