Pytorch使用笔记
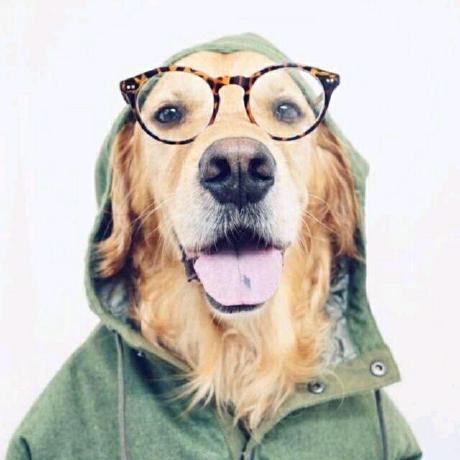
Pytorch有非常多的函数,这篇文章长期更新,记录使用到的函数。
参考资源:
Categorical
官方文档:https://pytorch.org/docs/stable/distributions.html
CLASS torch.distributions.categorical.Categorical(probs=None, logits=None, validate_args=None)
作用:创建一个以probs为参数的类别分布。
采样的样本是
如果probs是一维的,那么就是对对应的index进行采样。
如果probs是二维的,那么相当于对一批一维的数组进行采样。
Example:
1 | probs = torch.FloatTensor([[0.05, 0.1, 0.85], [0.05, 0.05, 0.9]]) |
nn.Linear
官方文档:https://pytorch.org/docs/stable/generated/torch.nn.Linear.html#torch.nn.Linear
CLASS torch.nn.Linear(in_features: int, out_features: int, bias: bool = True)
作用:对于输入的数据应用一个线性变换,
- 参数:in_features, out_features, bias
- 变量:Linear.weight,其shape是(out_features, in_features),注意到
, Linear.bias
nn.optim.Adam
官方文档:https://pytorch.org/docs/stable/optim.html
CLASS torch.optim.Adam(params, lr=0.001, betas=(0.9, 0.999), eps=1e-08, weight_decay=0, amsgrad=False)
torch.nn.functional
官方文档:https://pytorch.org/docs/stable/nn.functional.html
主要包含了:convolution函数,Pooling函数,非线性激活函数,Normalization函数,线性函数,Dropout函数,距离函数,损失函数,视觉函数
torch.nn.functional.softmax函数
官方文档:https://pytorch.org/docs/stable/nn.functional.html
torch.nn.functional.softmax(input: torch.Tensor, dim: Optional[int] = None, _stacklevel: int = 3, dtype: Optional[int] = None) → torch.Tensor
softmax函数定义:
对一个n维的输入Tensor执行softmax操作,输出一个n维的Tensor,将他们重新调整到和为1
torch.tensor
官方文档:https://pytorch.org/docs/stable/generated/torch.tensor.html#torch.tensor
torch.tensor(data, dtype=None, device=None, requires_grad=False, pin_memory=False) → Tensor
warning:
torch.tensor()
会复制data
,如果有一个Tensor
数据的话,并且想避免复制,可以使用torch.Tensor.requires_grad_()
,torch.Tensor.detach()
- 如果有一个numpy的
ndarray
并且想要避免复制,可以使用torch.as_tensor()
torch.tensor.detach
官方文档:https://pytorch.org/docs/stable/autograd.html#torch.Tensor.detach
从原有的计算图中分离出某一个tensor,这个新的tensor和原来的tensor是共用数据的,一者改变,另一者也会跟着改变,而且新分离得到的tensor的requires_grad=False
即不可求导的。
什么时候会用到detach
?当我们在训练网络的时候可能希望保持一部分的网络参数不变,只对其中一部分的参数进行调整;或者只训练部分分支网络,并不让其梯度对主网络的梯度造成影响,这时候我们就需要使用detach()函数来切断一些分支的反向传播。
注意:
- 即使之后重新将它的requires_grad设置为True,它也不会具有梯度grad
torch.exp
官方文档:https://pytorch.org/docs/stable/generated/torch.exp.html#torch.exp
torch.exp(input, out=None) → Tensor
给定一个输入Tensor,输出Tensor的每个元素是输入元素分别取exp
torch.log
官方文档:https://pytorch.org/docs/stable/generated/torch.log.html#torch.log
torch.log(input, out=None) → Tensor
给定一个输入Tensor,输出Tensor的每个元素是输入元素分别取log
torch.clamp
官方文档:https://pytorch.org/docs/stable/generated/torch.clamp.html#torch.clamp
torch.clamp(input, min, max, out=None) → Tensor
将一个输入的Tensor的每个元素限幅在min和max内,其中,min,max参数都是一个number。
torch.mm
官方文档:https://pytorch.org/docs/stable/generated/torch.mm.html#torch.mm
torch.mm(input, mat2, out=None) → Tensor
执行矩阵乘法
torch.squeeze
官方文档:https://pytorch.org/docs/stable/generated/torch.squeeze.html#torch.squeeze
torch.squeeze(input, dim=None, out=None) → Tensor
作用:降维
默认去除张量形状中的1。例如如果输入是(Ax1xBx1xCx1xD),那么输出形状就是(AxBxCxD)
torch.nn.functional.relu
官方文档:https://pytorch.org/docs/stable/generated/torch.nn.ReLU.html#torch.nn.ReLU
torch.gather
官方文档:https://pytorch.org/docs/stable/generated/torch.gather.html#torch.gather
torch.gather(input, dim, index, out=None, sparse_grad=False) → Tensor
使用时候遇到的坑
torch.nn.parameter.Parameter
Parameters是Tensor子类
官方文档:https://pytorch.org/docs/stable/generated/torch.nn.parameter.Parameter.html
使用的时候需要注意输入参数的维度:
nn.Parameter(torch.zeros(output_dim, input_dim))
,当使用nn.Linear
构造网络的时候,其中
- 标题: Pytorch使用笔记
- 作者: Oliver xu
- 创建于 : 2020-08-14 19:07:50
- 更新于 : 2025-07-03 21:07:46
- 链接: https://blog.oliverxu.cn/2020/08/14/Pytorch使用笔记/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。